MongoDB
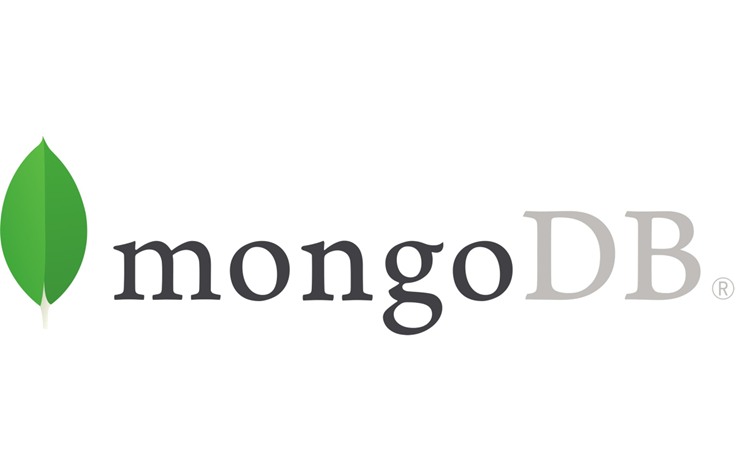
Introduction to MongoDB
MongoDB is a document-oriented, NoSQL database widely used for modern application development. It stores data in flexible, JSON-like documents, meaning fields can vary from document to document, and data structure can change over time. Its scalability, performance, and ease of use make it an ideal choice for handling large datasets and real-time data analytics.
MongoDB was designed to address the limitations of traditional relational databases. It is known for being schema-less, providing high availability, and allowing for horizontal scaling. Instead of storing data in rows and columns like traditional databases (SQL), MongoDB stores data as collections of documents. This makes it highly flexible and capable of handling a wide variety of data types.
What is MongoDB?
MongoDB is a document-oriented NoSQL database designed for scalability, flexibility, and performance. Developed by MongoDB Inc., it was first released in 2009 and has since become a cornerstone of many modern web applications and data-driven systems.
Key Features of MongoDB
1. Document-Oriented Storage
MongoDB uses a flexible schema to store data. It stores data in the form of BSON (Binary JSON), allowing for arrays, nested objects, and other complex data structures within a single document. Unlike traditional SQL databases, MongoDB doesn’t require predefined schemas, meaning that fields can be added, removed, or altered at any time without affecting the existing documents.
2. Scalability
MongoDB supports horizontal scaling through sharding. Sharding allows for distributing data across multiple servers, which improves both storage capacity and performance. MongoDB automatically manages the distribution of data across shards and balances load accordingly.
3. Indexing
To improve query performance, MongoDB supports various types of indexes, such as single field, compound, and geospatial indexes. These indexes help optimize searches within large datasets by quickly locating documents matching a query.
4. High Availability
MongoDB provides high availability through replication. Replica sets consist of two or more copies of data, ensuring data redundancy and failover support. If the primary node fails, the system automatically switches to a secondary node, minimizing downtime.
5. Aggregation Framework
MongoDB offers a powerful aggregation framework, allowing users to perform complex data transformations and analytics. It supports operations like filtering, grouping, sorting, and applying complex calculations, similar to SQL’s GROUP BY or JOIN operations.
6. Load Balancing
MongoDB has built-in load balancing that distributes read and write operations across replica sets, ensuring high throughput and reducing latency. This makes it suitable for handling high-traffic applications.
MongoDB Architecture
MongoDB uses a client-server architecture. The core components include:
- Documents: The primary unit of data in MongoDB, represented in BSON format.
- Collections: A grouping of documents, analogous to tables in relational databases. Collections don’t enforce schemas, so each document can have different fields.
- Databases: A logical container for collections, each with its own set of collections and documents.
- Shards: In a sharded cluster, data is distributed across multiple shards to support horizontal scaling.
- Replica Sets: A group of MongoDB instances that host the same data. Replica sets provide redundancy and high availability.
- Mongos: A routing service for sharded clusters that directs queries to the correct shards.
Setting Up MongoDB
- Download MongoDB from the official website.
- Install MongoDB following the instructions for your operating system.
- Start the MongoDB server using command: mongod
- Connect to MongoDB using the MongoDB shell: mong
CRUD Operations
Create Operations:
- Inserting a single document:
javascriptCopydb.users.insertOne({
name: “John Doe”,
age: 30,
email: “john@example.com”
})
- Inserting multiple documents:
javascriptCopydb.users.insertMany([
{ name: “Jane Smith”, age: 28, email: “jane@example.com” },
{ name: “Bob Johnson”, age: 35, email: “bob@example.com” }
])
3. Updating multiple documents:
javascriptCopydb.users.updateMany(
{ age: { $lt: 30 } },
{ $inc: { age: 1 } }
)
4. Delete Operations
Deleting a single document:
javascriptCopydb.users.deleteOne({ name: “John Doe” })
Deleting multiple documents:
javascriptCopydb.users.deleteMany({ age: { $gt: 50 } })
Regular expressions
db.users.find({ name: /^J/ })
Indexing in MongoDB
Indexes improve query performance:
javascriptCopy// Creating a single field index
db.users.createIndex({ age: 1 })
// Creating a compound index
db.users.createIndex({ name: 1, email: 1 })
// Creating a text index
db.articles.createIndex({ content: “text” })
MongoDB vs Redis: A Comprehensive Comparison for Optimization, Speed, Scalability, and Performance
When it comes to choosing a database for modern applications, two of the most commonly compared technologies are MongoDB and Redis. Both are highly regarded NoSQL databases that serve different use cases based on factors such as optimization, speed, scalability, and performance. This article provides a detailed comparison between MongoDB and Redis, helping developers and businesses decide which database suits their specific needs.
What is Redis?
Redis (Remote Dictionary Server) is an in-memory data structure store, often used as a key-value database, cache, and message broker. It supports different types of data structures like strings, lists, sets, and hashes. Redis is renowned for its lightning-fast speed since it primarily operates in-memory and offers advanced features like persistence, replication, and Lua scripting.
Type of Database
- MongoDB: A document-oriented NoSQL database that stores data in BSON (Binary JSON). It is designed for handling large volumes of unstructured or semi-structured data.
- Redis: An in-memory key-value store and cache that also supports other data structures like lists, sets, and hashes.
Speed and Performance
- MongoDB: Slower compared to Redis for read-heavy operations because MongoDB writes data to disk. However, MongoDB performs well with large datasets, especially when combined with indexes.
- Redis: Extremely fast because it operates entirely in memory, providing sub-millisecond latency. This makes Redis ideal for real-time applications like caching and session management.
Optimization
- MongoDB: Optimized for large-scale document storage and retrieval. It supports rich queries, complex aggregations, and offers flexibility for schema changes. Great for handling complex data models.
- Redis: Optimized for low-latency, high-throughput operations. It can be used for caching frequently accessed data, reducing load on a primary database. Redis also supports persistence with optional configuration for performance tuning.
Scalability
- MongoDB: Built for horizontal scaling via sharding, which distributes data across multiple servers. This allows MongoDB to handle large-scale applications with ease, supporting both high availability and distributed workloads.
- Redis: Supports horizontal scaling through clustering, where data is split across multiple Redis nodes. However, scaling Redis can be more complex because it stores everything in memory, meaning memory management is critical.
Data Persistence and Durability
- MongoDB: Persistence is a core feature, as MongoDB stores data on disk by default. It offers high durability with replication and journaling to ensure data integrity in case of crashes or failures.
- Redis: Primarily an in-memory database but offers AOF (Append-Only File) and RDB (Redis Database Backup) options for data persistence. While these options
make Redis more durable, it doesn’t match MongoDB’s out-of-the-box durability.
MongoDB vs MySQL: A Comparison
- Data Model:
- MongoDB: Document-oriented (NoSQL), stores data in BSON format.
- MySQL: Relational (SQL-based), uses tables with rows and columns.
- Schema:
- MongoDB: Flexible and schema-less, allowing dynamic data structures.
- MySQL: Fixed, predefined schema with strict data types and structure.
- Scalability:
- MongoDB: Supports horizontal scaling through sharding, distributing data across multiple servers.
- MySQL: Primarily scales vertically (by increasing server resources), with limited support for horizontal scaling.
- Joins:
- MongoDB: Limited support for joins; typically uses embedded documents and references for relationships.
- MySQL: Extensive support for complex joins and relationships between tables.
- Use Case:
- MongoDB: Ideal for real-time analytics, unstructured data, and flexible data models.
- MySQL: Best suited for structured data with complex relationships, where consistency is critical.
Advantages of MongoDB
- Flexible Schema: MongoDB’s schema-less nature allows developers to modify data structures without major downtime.
- Scalability: Horizontal scaling through sharding enables MongoDB to handle massive datasets efficiently.
- Powerful Aggregation Framework: Supports complex data operations and analytics.
- High Availability: Replication ensures data redundancy and failover support.
Disadvantages of MongoDB
- Performance with Complex Queries: While MongoDB excels in many areas, certain types of complex queries may not perform as well as traditional SQL databases.
- Memory Usage: MongoDB can be memory-intensive, especially when handling large datasets without proper indexing.
- Limited Transaction Support: Although MongoDB supports multi-document transactions, this feature is relatively new and may not be as mature as in relational databases.
Future Uses of MongoDB
With its ability to handle big data, real-time analytics, and IoT applications, MongoDB’s future is bright. It is widely used in sectors like e-commerce, social media, and healthcare, where fast data processing and scalability are critical. Its continuous development with features like enhanced transactions and better cloud integration ensures MongoDB will remain relevant for future application development.
Conclusion
MongoDB revolutionizes the way developers handle data, offering flexibility, scalability, and high availability for modern applications. While it has some limitations, especially in complex querying, its document-oriented approach, coupled with its horizontal scalability, makes MongoDB an excellent choice for handling dynamic and large-scale datasets. As technology evolves, MongoDB will continue to play a crucial role in shaping the future of data management.
References
- MongoDB Inc. “MongoDB Documentation.” mongodb.com
- Redis Labs. “Redis Documentation.” redis.io
- MySQL. “MySQL Documentation.” mysql.com
- Chodorow, Kristina. MongoDB: The Definitive Guide. O’Reilly Media, 2013.